Darkweb v2.0 public release is here
Lorem ipsum dolor sit amet, consectetur adipiscing elit lobortis arcu enim urna adipiscing praesent velit viverra sit semper lorem eu cursus vel hendrerit elementum morbi curabitur etiam nibh justo, lorem aliquet donec sed sit mi dignissim at ante massa mattis.
- Neque sodales ut etiam sit amet nisl purus non tellus orci ac auctor
- Adipiscing elit ut aliquam purus sit amet viverra suspendisse potent i
- Mauris commodo quis imperdiet massa tincidunt nunc pulvinar
- Adipiscing elit ut aliquam purus sit amet viverra suspendisse potenti
What has changed in our latest release?
Vitae congue eu consequat ac felis placerat vestibulum lectus mauris ultrices cursus sit amet dictum sit amet justo donec enim diam porttitor lacus luctus accumsan tortor posuere praesent tristique magna sit amet purus gravida quis blandit turpis.
All new features available for all public channel users
At risus viverra adipiscing at in tellus integer feugiat nisl pretium fusce id velit ut tortor sagittis orci a scelerisque purus semper eget at lectus urna duis convallis. porta nibh venenatis cras sed felis eget neque laoreet suspendisse interdum consectetur libero id faucibus nisl donec pretium vulputate sapien nec sagittis aliquam nunc lobortis mattis aliquam faucibus purus in.
- Neque sodales ut etiam sit amet nisl purus non tellus orci ac auctor
- Adipiscing elit ut aliquam purus sit amet viverra suspendisse potenti
- Mauris commodo quis imperdiet massa tincidunt nunc pulvinar
- Adipiscing elit ut aliquam purus sit amet viverra suspendisse potenti
Coding collaboration with over 200 users at once
Nisi quis eleifend quam adipiscing vitae aliquet bibendum enim facilisis gravida neque. Velit euismod in pellentesque massa placerat volutpat lacus laoreet non curabitur gravida odio aenean sed adipiscing diam donec adipiscing tristique risus. amet est placerat in egestas erat imperdiet sed euismod nisi.
โUt enim ad minim veniam, quis nostrud exercitation ullamco laboris nisi ut aliquip ex ea commodo consequat. Duis aute irure dolor in reprehenderit in voluptate velit esse cillumโ
Real-time code save every 0.1 seconds
Eget lorem dolor sed viverra ipsum nunc aliquet bibendum felis donec et odio pellentesque diam volutpat commodo sed egestas aliquam sem fringilla ut morbi tincidunt augue interdum velit euismod eu tincidunt tortor aliquam nulla facilisi aenean sed adipiscing diam donec adipiscing ut lectus arcu bibendum at varius vel pharetra nibh venenatis cras sed felis eget dolor cosnectur drolo.
Design patterns are essential for software development, providing a framework to create reliable, efficient, and maintainable software architectures. This blog post will discuss the top 5 Java design patterns: Singleton, Factory Method, Observer, Strategy, and Builder. We'll review each pattern's purpose, structure, participants, and collaborations and describe their benefits with practical examples. By the end of this post, readers will better understand how to use design patterns in their projects and further explore design patterns in greater detail.
Introduction to Design Patterns
Design patterns allow software engineers to solve common problems in their code and can be used as a library of best practices. They are described in terms of purpose, structure, participants, collaborations, and benefits. With design patterns, software engineers can create more maintainable and reusable code that other developers can easily understand and use. Design patterns can be categorized into three main types: creational, structural, and behavioral. Creational design patterns focus on creating objects while keeping the system flexible. Structural design patterns are concerned with combining classes or objects to form larger structures. Behavioral design patterns focus on how classes or objects interact with one another to achieve a certain goal.
The goal of using design patterns is to make code more maintainable and reusable by providing a framework for software engineers to follow when building applications. By creating solutions for common problems that software engineers may encounter when developing applications, design patterns help ensure that the resulting code is efficient and reliable.
By understanding how design patterns work and recognizing when they should be used in your projects, you can create better applications faster than ever! For readers who want to dig deeper into this topic, plenty of information is available online about different types of design pattern implementations and related topics such as object-oriented programming (OOP) principles and SOLID architecture principles.
Singleton Pattern
Imagine a government's central intelligence agency, like the CIA or MI6. There's only one such agency in a country, serving as the central point for all intelligence operations, similar to how a Singleton class provides a single point of access in software.
โ

โ
The Singleton Pattern is one of Java's most popular software design patterns. It restricts the instantiation of a class to one object and controls its creation, ensuring that only one instance of an object exists in memory. The Singleton Pattern is best suited when multiple program parts need to access a single shared resource. It is also useful for global data access or coordination among multiple classes. The basic idea behind the Singleton Pattern is that it allows you to control the number of instances created from a particular class. This is done by making sure that there is only ever one instance of a class in existence at any given time. You need to use private constructors and static methods or variables to implement this pattern.
To create a Singleton in Java, you must first define your class and make sure the constructor remains private so that it cannot be instantiated outside of its definition. After defining your class, you must create a static field referencing your singleton's instance. Finally, you must define a static method that returns an instance of your singleton class each time it is called upon - this method should also check if an instance already exists before creating another one to avoid duplication.
Using the Singleton Pattern, developers can maintain control over their objects' lifecycles and ensure they remain thread-safe while being used simultaneously across multiple threads and processes. Additionally, this pattern eliminates problems with duplicate resources and potential memory leaks due to incorrect usage or improper clean-up after completing object creation. With these benefits in mind, it becomes clear why many developers choose this pattern over other software design options available in Java today.
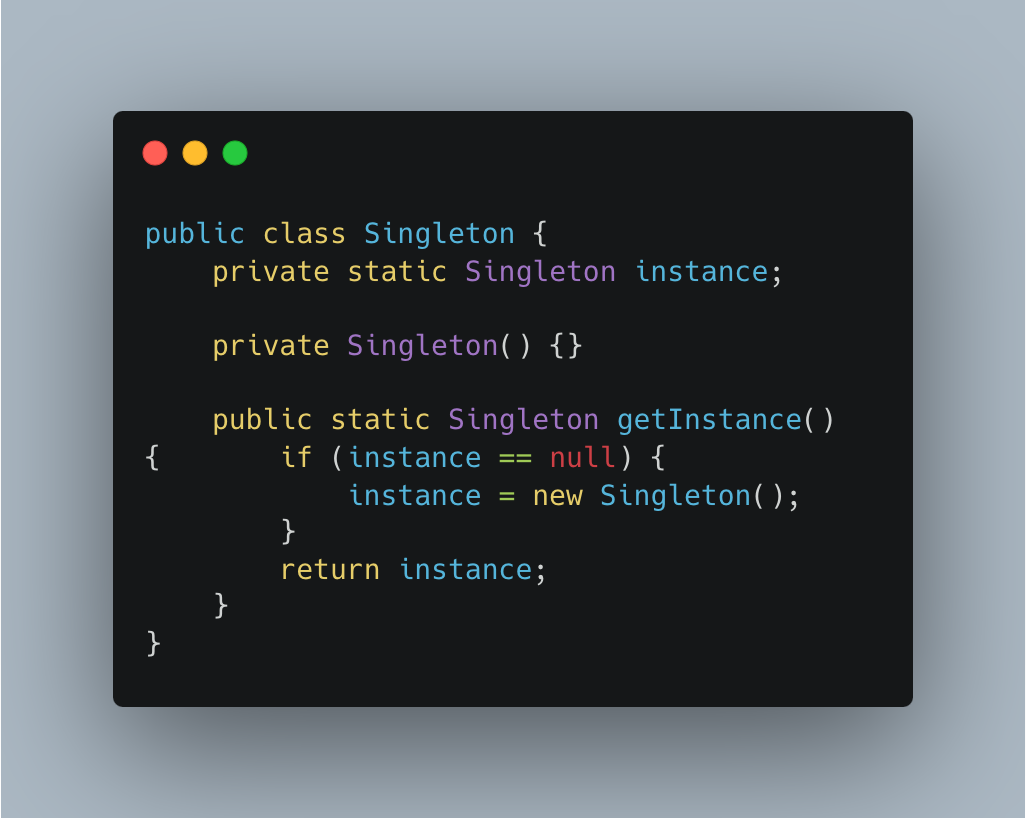
Factory Method Pattern
Imagine a construction contractor who builds different types of buildings (houses, offices, etc.). The contractor (the factory method) decides what type of building to construct based on the requirements, akin to how the Factory Method in software creates different objects based on input or configuration.
โ

โ
It's no secret that the Factory Method Pattern is a powerful tool for software development. This pattern provides an efficient way to decouple client code from the instantiation process, meaning developers can create objects without knowing which concrete class they need to initialize. It also takes advantage of inheritance and polymorphism, as subclasses are responsible for implementing the factory method. The Factory Method Pattern has multiple advantages over other object-creation techniques. Firstly, it eliminates the need for multiple constructors with different parameters, thus making coding simpler and reducing potential errors from incorrect parameter ordering. Secondly, this pattern promotes loose coupling between classes by keeping object creation separate from usage; this simplifies maintenance and enhances extensibility when introducing new types of objects.
However, consider some drawbacks before using this pattern in your project. For instance, debugging may become harder because all objects are created through abstract interfaces instead of their respective classes. In addition, if you wish to add a new type of object using this pattern, you may have to modify multiple files instead of a single one, as some other object creation techniques allow you to do.
Ultimately, the Factory Method Pattern is an excellent choice for many software projects. It offers flexibility when dealing with changing requirements or implementing new features into existing applications while allowing developers to create objects without fully understanding which concrete class they must instantiate.

Observer Pattern
Imagine a news subscription service. When there's breaking news (a state change), the news service (the subject) notifies all subscribers (observers) who have signed up to receive updates. This is similar to how the Observer pattern notifies all registered components when a particular event occurs in software.
โ
The Observer Pattern is a versatile and powerful behavioral design pattern that allows objects to be notified of changes in another object. This one-to-many dependency simplifies communication between multiple objects, as each observer receives notifications when the subject object changes state. It can also reduce memory usage by enabling delayed initialization - observers are only instantiated when needed, not for every object instance. The Observer Pattern offers several advantages over other designs; it decouples the observer classes from the subject class, making it easier to maintain and extend existing code without affecting other parts of the program. However, developers should ensure its successful implementation as an event-driven development approach may be necessary for managing events efficiently and avoiding performance issues due to too many observers in the system. Unit tests can help identify potential bugs before deployment, while libraries like RxJava or EventBus can simplify communications between components while ensuring optimal performance.
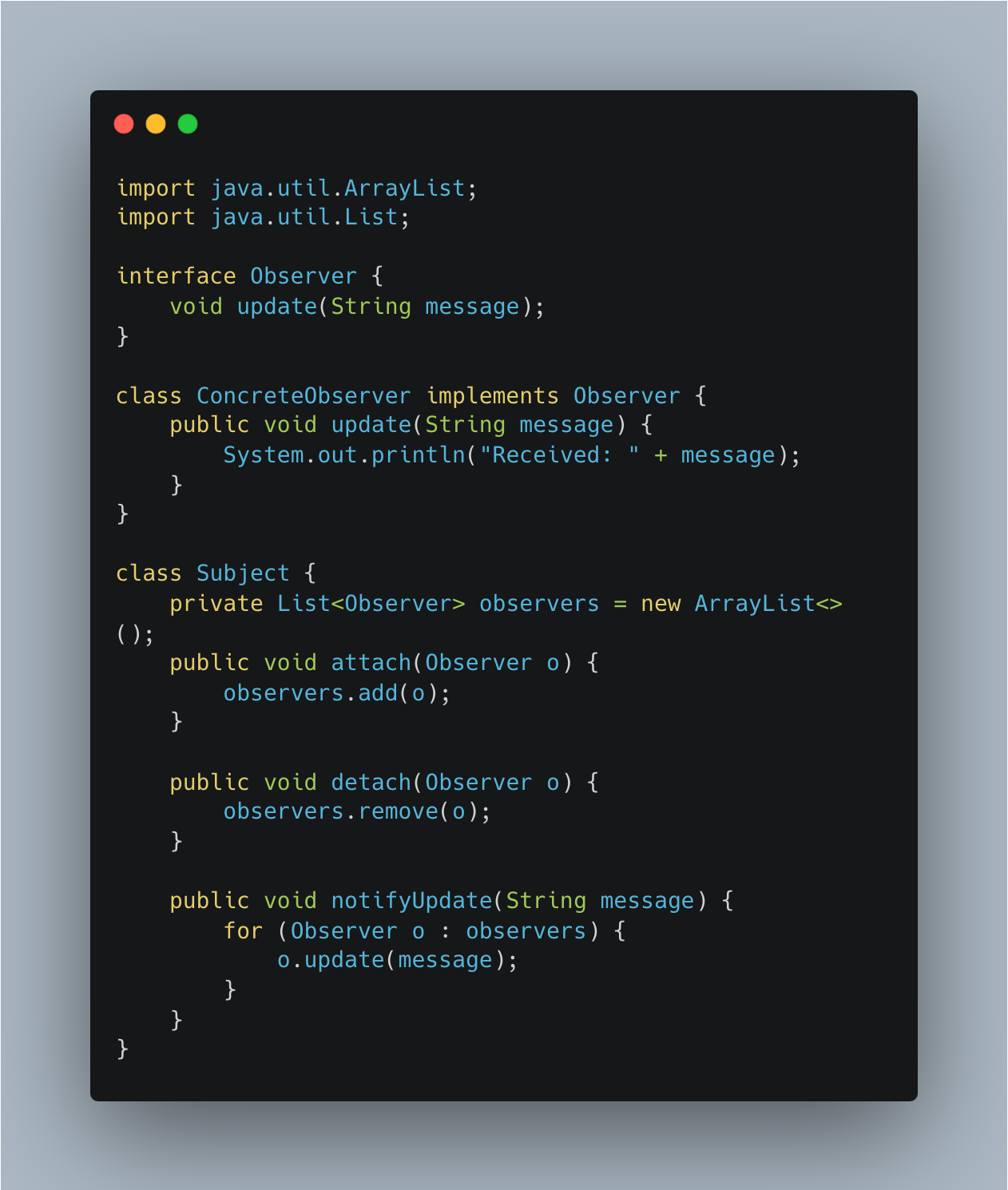
Strategy Pattern
Imagine a travel planner who chooses different modes of transportation (car, train, airplane) based on the destination, budget, and time. Each mode of transport is a strategy, and the planner (context) selects the appropriate one. Similarly, the Strategy pattern selects the most suitable algorithm based on the context.
โ
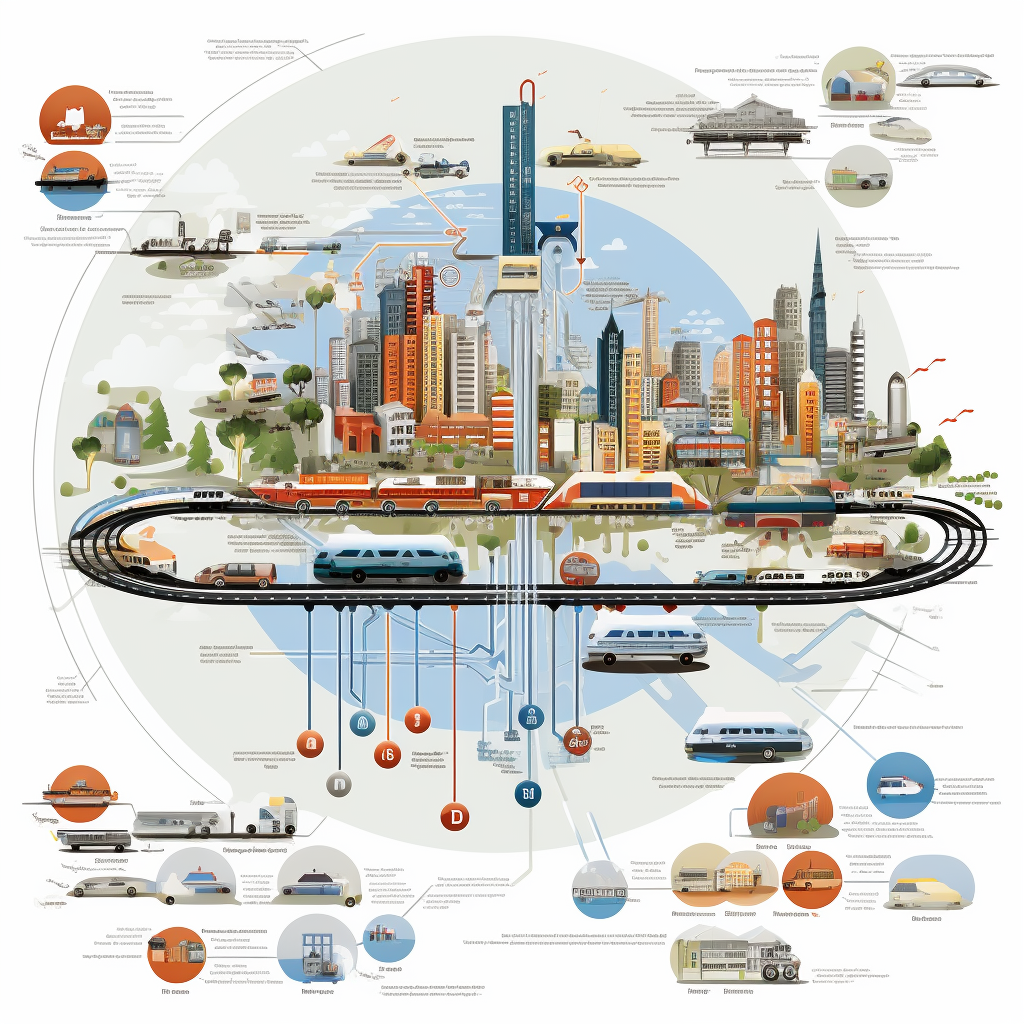
โ
The Strategy Pattern is a valued resource for software engineers, permitting them to develop objects that can be used in varied scenarios and dynamically change their conduct during runtime. This pattern defines a collection of algorithms, encapsulates them, and makes them interchangeable. By employing the Strategy Pattern, developers can reduce the number of classes while still having the capacity to switch between strategies as needed. One application of the Strategy Pattern is in gaming development. For example, a game may have different levels with diverse enemy AI patterns. With this pattern employed, developers can package these patterns into separate classes and then transition between them based on their playing level. Consequently, players will encounter distinct challenges depending on their chosen difficulty level without requiring additional code written for each situation.
Moreover, there are numerous other benefits associated with utilizing this pattern, such as simplifying complexity by abstracting away details from client code, advancing maintainability by making it simpler to add new algorithms or modify existing ones, and improving flexibility by enabling developers to rapidly exchange one algorithm for another without having to refactor large parts of their codebase.
Nevertheless, there are some drawbacks linked with this pattern, too - especially that it adds an extra layer of complexity to your software design that might not be necessary if only one type of algorithm or behavior is needed. In addition, if you have multiple strategies with similar logic but differ slightly in implementation details - such as two sorting algorithms - you would need to write individual classes for each rather than reusing analogous components across plans.
Ultimately, the Strategy Pattern presents a great approach for developers to simplify complex applications and make their code more flexible and maintainable in the long run. It allows engineers to switch behaviors at runtime without needing comprehensive refactoring whenever they want to alter how something works under particular conditions or contexts. With wise consideration and comprehension of its advantages and boundaries, this pattern can help many teams significantly enhance their development processes.

Builder Pattern
ย Imagine a meal at a fast-food restaurant where you can customize your burger, drink, and side. The builder (staff) assembles your meal based on your choices (components). In software, the Builder pattern constructs complex objects step by step, allowing different representations or configurations.
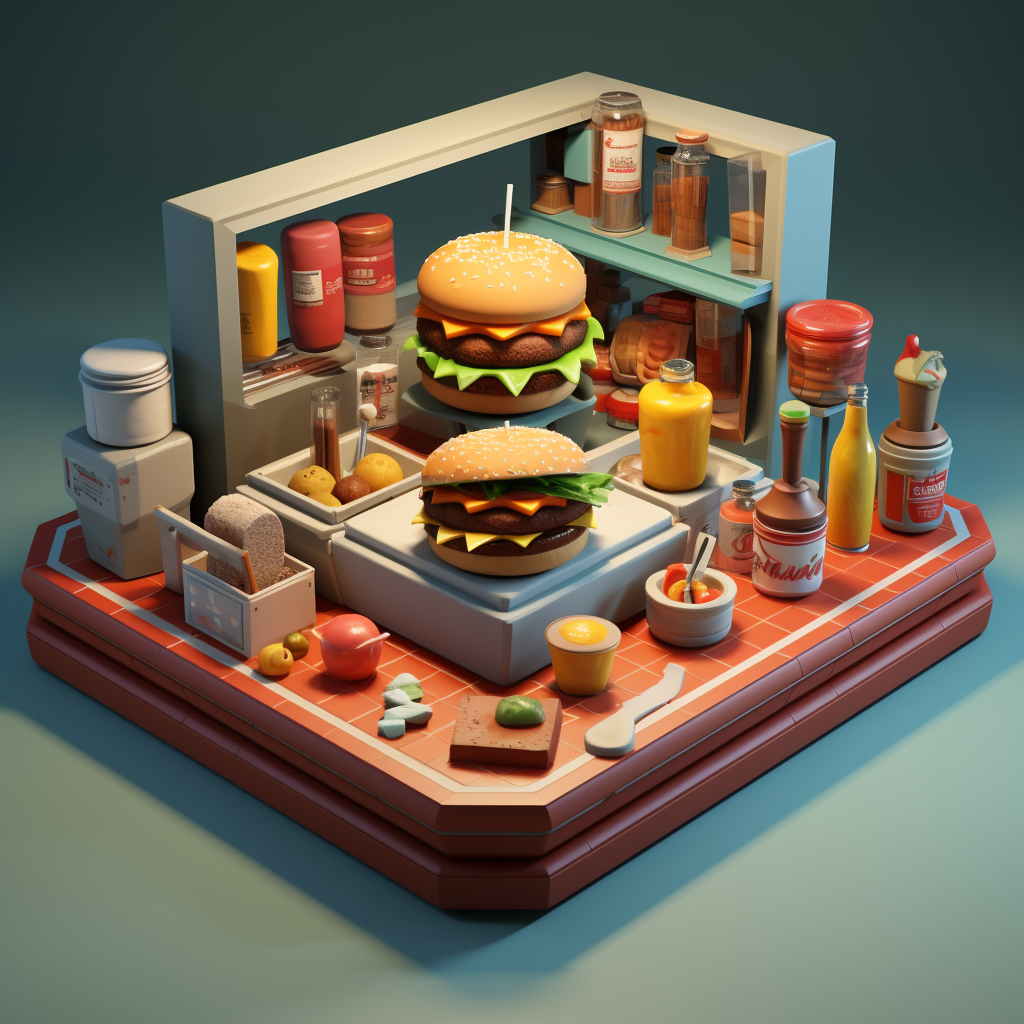
The Builder Pattern is an essential creational design pattern for software engineering, providing developers with a consistent method of constructing complex objects. It allows the construction process to be separated from its representation so that different types of representations can be created using the same instructions. This reduces complexity and minimizes errors when constructing complex objects with many parameters. To use this pattern effectively, there are four main components: builder, director, product, and client. The builder sets up an interface for constructing parts that are assembled into a whole product, while the director uses this interface to control how these parts are assembled into larger structures; finally, the product represents what's built by both builders and directors. All three components need to collaborate for it to work correctly as each part can be designed independently without affecting any other parts, which increases flexibility during development and testing stages as well as makes it easier to understand code since all related operations are grouped together in one place instead scattered throughout various classes or modules.
Benefits of using the Builder Pattern include reducing duplicate code within your project since you don't need multiple constructors for different types of objects; it also helps make debugging easier because all necessary information about an object resides within one class, which eliminates problems caused by incorrect usage or unexpected values; lastly, it simplifies maintenance because developers only need to modify code in one place if something changes instead having to update multiple classes or modules across their project.
Due to its versatility and effectiveness at allowing developers to create complex objects with minimal errors while maintaining readability and flexibility during development stages as well as later on when performing maintenance tasks on existing projects, understanding the Builder Pattern is essential for any Java developer looking for ways to optimize their workflow without compromising quality.
.png)
Conclusion
When it comes to software engineering, design patterns can be incredibly useful for setting up and managing complex systems. The five Java Design Patterns discussed in this article โ Singleton, Factory Method, Observer, Strategy, and Builder โ offer different ways to solve common development problems. Each pattern offers its own unique benefits and drawbacks that need to be taken into consideration before implementation. Learning about design patterns is essential for any Java developer looking to optimize their workflow without compromising quality. By understanding the purpose, structure, participants, collaborations, and benefits of each pattern discussed here, developers can make more informed decisions when creating objects or designing systems with complex requirements. Additionally, they should use existing libraries such as RxJava or EventBus to simplify communications between components further while ensuring optimal performance.
This blog post has provided readers with an introduction to some of the most popular Java Design Patterns. If readers are looking for further exploration of design patterns or related topics, such as event-driven development approaches or optimizing communication between components, then there are plenty of resources online for them to explore. To get started on learning more about these design patterns and other related topics, we suggest reading 'Head First Design Patterns' by Eric Freeman et al., 'Design Patterns Explained Simply' by Alexey Zimarev, or the official Oracle documentation on Java Design Patterns.'