In the intricate and unpredictable realm of software development, particularly within Java frameworks, exception testing emerges as a vital shield against the unforeseen. Just as a seasoned sailor prepares for turbulent seas, exception testing equips Java developers with the tools to navigate through the stormy waters of errors, invalid inputs, and unexpected scenarios. This critical component of Java unit testing ensures that your code not only meets its functional requirements but also possesses the resilience to withstand and gracefully handle situations that deviate from the norm. By integrating exception testing into your development process, using tools like @Test(expected = ExceptionClass.class) annotation and assertThrows() method in JUnit, you fortify your application against potential failures, ensuring a robust and reliable end product.
Exception testing in Java can be likened to a seasoned mountain guide preparing for an expedition. Just as the guide anticipates and plans for various adverse conditions, such as sudden weather changes, avalanches, or unexpected terrain challenges, exception testing involves preparing your code for unforeseen circumstances. The guide's meticulous planning, knowledge of the terrain, and ability to adapt quickly to unexpected events are akin to a developer's use of specific annotations and assert methods in Java to predict and manage potential errors. Both the mountain guide and the Java developer must be proactive, anticipate potential risks, and have a strategy in place to handle these challenges effectively to ensure a safe and successful journey.
Exception testing: handling the unexpected in Java
Exception testing is a critical part of any Java unit testing strategy. It allows you to verify that your code behaves as expected when encountering exceptional circumstances, such as errors, invalid inputs, or unexpected conditions. By writing exception tests, you can ensure that your code is robust, reliable, and able to handle unexpected situations gracefully. To test for specific exceptions, you can use the `@Test(expected = ExceptionClass.class)` annotation in JUnit. This annotation tells JUnit to expect a specific exception to be thrown when the test method is executed. If the expected exception is not thrown, the test will fail.
You can also use try-catch blocks to catch and handle exceptions in your code. This allows you to perform specific actions when an exception occurs, such as logging the error or displaying a user-friendly message.
To assert that the correct exception is thrown when expected, you can use the `assertThrows()` method in JUnit. This method takes two arguments: the expected exception class and a lambda expression that represents the code that you expect to throw the exception. If the expected exception is not thrown, the test will fail.
Finally, you can also verify that the exception message is accurate and informative. This can be done by using the `assertEquals()` method to compare the actual exception message with the expected message.
Example
Let's create a simple code example in Java to demonstrate exception testing using JUnit. We will create a class Calculator with a method divide, which throws an ArithmeticException when attempting to divide by zero. We'll then write a JUnit test to ensure that the exception is correctly thrown.
First, the Calculator class:
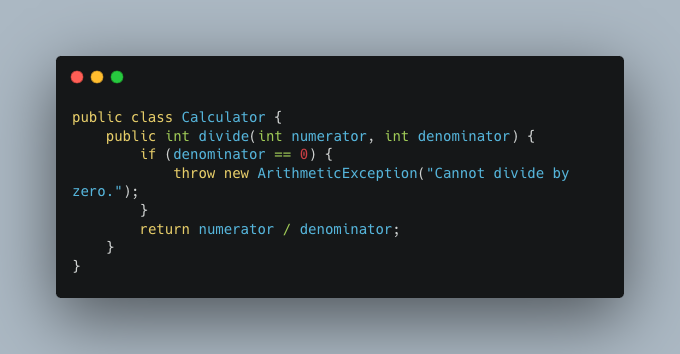
Now, let's write a JUnit test class CalculatorTest to test the divide method:

In this JUnit test class, there are three test methods:
- testDivideByZero: This test expects an ArithmeticException when calling divide with a zero denominator. It uses the @Test(expected = ArithmeticException.class) annotation to specify the expected exception.
- testDivideNormal: This test checks the normal behavior of the divide method when the input is valid.
- testDivideExceptionMessage: This test checks the exception message to ensure it's informative and correct. It uses a try-catch block to catch the exception and then asserts that the message is as expected. If no exception is thrown, fail() is called to indicate the test should fail.
This example demonstrates how to write exception tests in Java using JUnit, ensuring that the code behaves correctly in both normal and exceptional circumstances.
By following these tips, you can write robust code that is able to handle unexpected exceptions gracefully. This will help you to ensure the quality and reliability of your Java applications. The practice of exception testing in Java is akin to an essential survival skill in the wilderness of software development. It's about anticipating the unpredictable, preparing for it, and then navigating through it with confidence and control. By employing techniques such as the @Test(expected = ExceptionClass.class) annotation, try-catch blocks, assertThrows() method, and validating exception messages, developers can create Java applications that not only perform under normal conditions but also exhibit grace and resilience when faced with the unexpected. This approach is crucial for building high-quality, reliable software that can stand the test of time and the unpredictability of real-world applications.